|
|
|

|
|
|
|
|
|

|
|
 |
Clocks
|
|
|
Clocks are special objects in SystemC. They are used for generating clocks, that are used for synchronizing the events in SystemC models link in any other HDL. |
|
|

|
|
|
A clock object has a number of data members to store clock settings, and methods to perform clock actions. To create a clock object use the following syntax: |
|
|

|
|
|
sc_clock clock("my_clock", 10, 0.5, 1, false); |
|
|

|
|
|
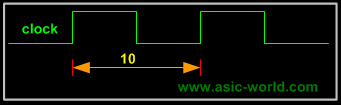 |
|
|

|
|
|
This declaration will create a clock object named clock with a period of 10 time units, a duty cycle of 50%, the first edge will occur at 1 time units, and the first value will be false. All of these arguments have default values except for the clock name. The period defaults to 1, the duty cycle to 0.5, the first edge to 0, and the first value to true. |
|
|

|
|
|
Typically clocks are created at the top level of the design in the testbench and passed down through the module hierarchy to the rest of the design. |
|
|

|
|
|
When binding the clock to a port the designer must use the clock signal generated by the clock object to map to a port. This done by using the signal method of the clock object. |
|
|

|
|
|
For SC_CTHREAD processes the clock object is directly mapped to the clock input of the process and the signal() method is not required. |
|
|

|
|
|
|
|
|

|
|
 |
Example : Clocks
|
|
|
Below example is same as what we saw in last page, only difference is we have replaced the manual clock with sc_clock signal and used clock.signal() method at port binding. |
|
|

|
|
|
1 #include <systemc.h>
2
3 SC_MODULE (some_block) {
4 sc_in<bool> clock;
5 sc_in<sc_bit> data;
6 sc_in<sc_bit> reset;
7 sc_in<sc_bit> inv;
8 sc_out<sc_bit> out;
9
10 void body () {
11 if (reset.read() == 1) {
12 out = sc_bit(0);
13 } else if (inv.read() == 1) {
14 out = ~out.read();
15 } else {
16 out.write(data.read());
17 }
18 }
19
20 SC_CTOR(some_block) {
21 SC_METHOD(body);
22 sensitive << clock.pos();
23 }
24 };
25
26
27 SC_MODULE (signal_bind) {
28 sc_in<bool> clock;
29
30 sc_signal<sc_bit> data;
31 sc_signal<sc_bit> reset;
32 sc_signal<sc_bit> inv;
33 sc_signal<sc_bit> out;
34 some_block *block;
35 int done;
36
37 void do_test() {
38 while (true) {
39 wait();
40 if (done == 0) {
41 cout << "@" << sc_time_stamp() <<" Starting test"<<endl;
42 wait();
43 wait();
44 inv = sc_bit('0');
45 data = sc_bit('0');
46 cout << "@" << sc_time_stamp() <<" Driving 0 all inputs"<<endl;
47 // Assert reset
48 reset = sc_bit('1');
49 cout << "@" << sc_time_stamp() <<" Asserting reset"<<endl;
50 // Deassert reset
51 wait();
52 wait();
53 reset = sc_bit('0');
54 cout << "@" << sc_time_stamp() <<" De-Asserting reset"<<endl;
55 // Assert data
56 wait();
57 wait();
58 cout << "@" << sc_time_stamp() <<" Asserting data"<<endl;
59 data = sc_bit('1');
60 wait();
61 wait();
62 cout << "@" << sc_time_stamp() <<" Asserting inv"<<endl;
63 inv = sc_bit('1');
64 wait();
65 wait();
66 cout << "@" << sc_time_stamp() <<" De-Asserting inv"<<endl;
67 inv = sc_bit('0');
68 wait();
69 wait();
70 done = 1;
71 }
72 }
73 }
74
75 void monitor() {
76 cout << "@" << sc_time_stamp() << " data :" << data
77 << " reset :" << reset << " inv :"
78 << inv << " out :" << out <<endl;
79 }
80
81 SC_CTOR(signal_bind) {
82 block = new some_block("SOME_BLOCK");
83 block->clock (clock) ;
84 block->data (data) ;
85 block->reset (reset) ;
86 block->inv (inv) ;
87 block->out (out) ;
88 done = 0;
89
90 SC_CTHREAD(do_test,clock.pos());
91 SC_METHOD(monitor);
92 sensitive << data << reset << inv << out;
93 }
94
95 };
96
97
98 int sc_main (int argc, char* argv[]) {
99 sc_clock clock ("my_clock",1,0.5);
100
101 signal_bind object("SIGNAL_BIND");
102 object.clock (clock.signal());
103 sc_trace_file *wf = sc_create_vcd_trace_file("clock");
104 sc_trace(wf, object.clock, "clock");
105 sc_trace(wf, object.reset, "reset");
106 sc_trace(wf, object.data, "data");
107 sc_trace(wf, object.inv, "inv");
108 sc_trace(wf, object.out, "out");
109
110 sc_start(100);
111 sc_close_vcd_trace_file(wf);
112
113 cout<<"Terminating Simulation"<<endl;
114 return 0;// Terminate simulation
115 }
You could download file clock.cpp here
|
|
|

|
|
 |
Simulation Output : Clocks
|
|
|

|
|
|
SystemC 2.0.1 --- Oct 6 2006 19:17:37
Copyright (c) 1996-2002 by all Contributors
ALL RIGHTS RESERVED
@0 s data :0 reset :0 inv :0 out :0
WARNING: Default time step is used for VCD tracing.
@1 ns Starting test
@3 ns Driving 0 all inputs
@3 ns Asserting reset
@3 ns data :0 reset :1 inv :0 out :0
@5 ns De-Asserting reset
@5 ns data :0 reset :0 inv :0 out :0
@7 ns Asserting data
@7 ns data :1 reset :0 inv :0 out :0
@8 ns data :1 reset :0 inv :0 out :1
@9 ns Asserting inv
@9 ns data :1 reset :0 inv :1 out :1
@10 ns data :1 reset :0 inv :1 out :0
@11 ns De-Asserting inv
@11 ns data :1 reset :0 inv :0 out :1
Terminating Simulation
|
|
|

|
|
|

|
|
|

|
|
|
|
|
|

|
|
|
|
|
|

|
|
Copyright © 1998-2025 |
Deepak Kumar Tala - All rights reserved |
Do you have any Comment? mail me at:deepak@asic-world.com
|
|