|
|
|

|
|
|
|
|
|

|
|
 |
Introduction
|
|
|
If you refer to any book on programming language it starts with "hello World" program, once you have written the program, you can be sure that you can do something in that language . |
|
|

|
|
|
Well I am also going to show how to write a "hello world" program in VHDL, followed by "counter" design in VHDL. |
|
|

|
|
 |
Hello World Program
|
|
|

|
|
|
1 entity hello_world is
2 end;
3
4 architecture hello_world of hello_world is
5 begin
6 stimulus : process
7 begin
8 assert false report "Hello World By Deepak"
9 severity note;
10 wait;
11 end process stimulus;
12 end hello_world;
You could download file hello_world.vhd here
|
|
|

|
|
|
Words in green are comments, blue are reserved words, Any program in VHDL starts with reserved word entity <entity_name>, In the above example line 8 contains entity hello_world. |
|
|

|
|
|
Line 4 contains architecture hello_world for entity hello_world, Architecture is body for entity, which implements the functionality for entity. |
|
|

|
|
 |
Hello World Program Output
|
|
|

|
|
|
Hello World by Deepak
|
|
|

|
|
|
|
|
|

|
|
 |
Counter Design Block
|
|
|

|
|
|
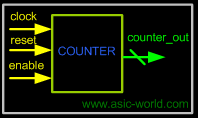 |
|
|

|
|
 |
Counter Design Specs
|
|
|

|
|
|
- 4-bit synchronous up counter.
- active high, synchronous reset.
- Active high enable.
|
|
|

|
|
 |
Counter Design
|
|
|

|
|
|
1 library ieee ;
2 use ieee.std_logic_1164.all;
3 use ieee.std_logic_unsigned.all;
4
5 entity counter is
6 port( clk: in std_logic;
7 reset: in std_logic;
8 enable: in std_logic;
9 count: out std_logic_vector(3 downto 0)
10 );
11 end counter;
12
13 architecture behav of counter is
14 signal pre_count: std_logic_vector(3 downto 0);
15 begin
16 process(clk, enable, reset)
17 begin
18 if reset = '1' then
19 pre_count <= "0000";
20 elsif (clk='1' and clk'event) then
21 if enable = '1' then
22 pre_count <= pre_count + "1";
23 end if;
24 end if;
25 end process;
26 count <= pre_count;
27 end behav;
You could download file counter.vhd here
|
|
|

|
|
 |
Counter Test Bench
|
|
|
Any digital circuit, no matter how complex, needs to be tested. For the counter logic, we need to provide a clock and reset logic. Once the counter is out of reset, we toggle the enable input to the counter, and check the waveform to see if the counter is counting correctly. The same is done in VHDL |
|
|

|
|
|
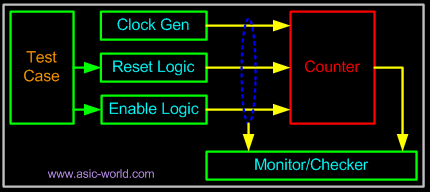 |
|
|

|
|
|
Counter testbench consists of clock generator, reset control, enable control and monitor/checker logic. Below is the simple code of testbench without the monitor/checker logic. |
|
|

|
|
|
1 library ieee ;
2 use ieee.std_logic_1164.all;
3 use ieee.std_logic_unsigned.all;
4 use ieee.std_logic_textio.all;
5 use std.textio.all;
6
7 entity counter_tb is
8 end;
9
10 architecture counter_tb of counter_tb is
11
12 component counter
13 port ( count : out std_logic_vector(3 downto 0);
14 clk : in std_logic;
15 enable: in std_logic;
16 reset : in std_logic);
17 end component ;
18
19 signal clk : std_logic := '0';
20 signal reset : std_logic := '0';
21 signal enable : std_logic := '0';
22 signal count : std_logic_vector(3 downto 0);
23
24 begin
25
26 dut : counter
27 port map (
28 count => count,
29 clk => clk,
30 enable=> enable,
31 reset => reset );
32
33 clock : process
34 begin
35 wait for 1 ns; clk <= not clk;
36 end process clock;
37
38 stimulus : process
39 begin
40 wait for 5 ns; reset <= '1';
41 wait for 4 ns; reset <= '0';
42 wait for 4 ns; enable <= '1';
43 wait;
44 end process stimulus;
45
46 monitor : process (clk)
47 variable c_str : line;
48 begin
49 if (clk = '1' and clk'event) then
50 write(c_str,count);
51 assert false report time'image(now) &
52 ": Current Count Value : " & c_str.all
53 severity note;
54 deallocate(c_str);
55 end if;
56 end process monitor;
57
58 end counter_tb;
You could download file counter_tb.vhd here
|
|
|

|
|
|
time clk reset enable counter
0 1 0 0 xxxx
5 0 1 0 xxxx
10 1 1 0 xxxx
11 1 1 0 0000
15 0 0 0 0000
20 1 0 0 0000
25 0 0 1 0000
30 1 0 1 0000
31 1 0 1 0001
35 0 0 1 0001
40 1 0 1 0001
41 1 0 1 0010
45 0 0 1 0010
50 1 0 1 0010
51 1 0 1 0011
55 0 0 1 0011
60 1 0 1 0011
61 1 0 1 0100
65 0 0 1 0100
70 1 0 1 0100
71 1 0 1 0101
75 0 0 1 0101
80 1 0 1 0101
81 1 0 1 0110
85 0 0 1 0110
90 1 0 1 0110
91 1 0 1 0111
95 0 0 1 0111
100 1 0 1 0111
101 1 0 1 1000
105 0 0 1 1000
110 1 0 1 1000
111 1 0 1 1001
115 0 0 1 1001
120 1 0 1 1001
121 1 0 1 1010
125 0 0 0 1010
|
|
|

|
|
 |
Counter Waveform
|
|
|

|
|
|
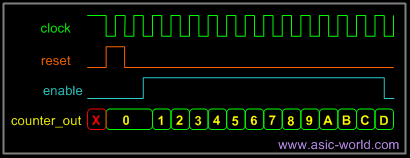 |
|
|

|
|
|

|
|
|

|
|
|
|
|
|

|
|
|
|
|
|

|
|
Copyright © 1998-2025 |
Deepak Kumar Tala - All rights reserved |
Do you have any Comment? mail me at:deepak@asic-world.com
|
|