|
|
|

|
|
|
|
|
|

|
|
 |
vmm_xactor_callback
|
|
|
Callbacks in general are used to executed a user defined method of predefined name when certain event happens in BFM or any class of interest. |
|
|

|
|
|
Some of the applications of callbacks are |
|
|

|
|
|
- Before sending CRC field, so callback, in callback user can decide to inject CRC in frame
- Once a frame is received, do callback, in callback user can decide if he wants to send flow control frame
- Once a frame is received, do callback, in callback decide if frame needs to be dropped
|
|
|

|
|
|
For any kind of callback implementation, one needs base class of callback. In vmm this class name is vmm_xactor_callback. |
|
|
To use this class following steps needs to be followed |
|
|

|
|
|
- Extend vmm_xactor_callback class and create my_callback class (You can have any name)
- Define standard virtual methods (Example my_cb) which will be executed by your bfm class
- User need to extend my_callback class (example my_abc_callback) and create body of standard virtual methods (my_cb)
- Create instance of this new clas (my_abc_callback)
- Add this new class instance to vmm_xactor class with method append_callback
|
|
|

|
|
|

|
|
|
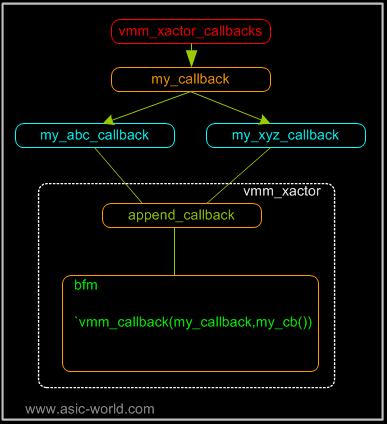 |
|
|

|
|
|

|
|
|
|
|
|

|
|
 |
Example : vmm_xactor_callback
|
|
|

|
|
|
1 `include "vmm.sv"
2
3 class my_data extends vmm_data;
4 vmm_log log;
5 int corrupt_crc;
6 int crc;
7 int addr;
8 int data;
9 int drop;
10 function new(vmm_log log);
11 super.new(log);
12 this.log = log;
13 this.corrupt_crc = 0;
14 this.crc = 0;
15 this.addr = 0;
16 this.data = 0;
17 this.drop = 0;
18 endfunction
19 endclass
20
21 // Need to extend from base class vmm_xactor_callback
22 virtual class my_callback extends vmm_xactor_callbacks;
23 vmm_log log;
24
25 function new(vmm_log log);
26 super.new();
27 this.log = log;
28 endfunction
29
30 virtual function my_cb(ref my_data my_d);
31 `vmm_warning(this.log,"Nothing is implemented here");
32 endfunction
33
34 endclass
35
36 // Need to extend from base class my_callback
37 class my_abc_callback extends my_callback;
38 int cnt;
39 function new(vmm_log log);
40 super.new(log);
41 cnt = 0;
42 endfunction
43
44 virtual function my_cb(ref my_data my_d);
45 cnt ++;
46 if (cnt %2 == 0) begin
47 `vmm_warning(this.log,"Corrupting CRC");
48 my_d.corrupt_crc = 1;
49 end
50 endfunction
51 endclass
52
53 // Need to extend from base class my_callback
54 class my_xyz_callback extends my_callback;
55 int cnt;
56 function new(vmm_log log);
57 super.new(log);
58 cnt = 0;
59 endfunction
60
61 virtual function my_cb(ref my_data my_d);
62 cnt ++;
63 if (cnt %2 == 1) begin
64 `vmm_warning(this.log,"Doing nothing");
65 end
66 endfunction
67 endclass
68
69 // Need to extend from base class vmm_xactor
70 class eth_tx extends vmm_xactor;
71 vmm_log log;
72
73 function new(vmm_log log,string name);
74 super.new(name, "parent");
75 this.log = log;
76 endfunction
77
78 virtual task main();
79 super.main();
80 fork
81 begin
82 this.bfm();
83 end
84 join_none
85 endtask
86
87 task bfm();
88 my_data mdata;
89 while(1) begin
90 #10 ; // This can be clock event
91 wait_if_stopped();
92 mdata = new(log);
93 `vmm_note(log,"Ethernet transmit bfm is running");
94 #10 ; // Lets execute callback
95 `vmm_callback(my_callback,my_cb(mdata));
96 if (mdata.corrupt_crc) begin
97 `vmm_warning(this.log,"Corrupting CRC on user request");
98 end
99 end
100 endtask
101 endclass
102
103 program test();
104 vmm_log log = new("test","test");
105 eth_tx tx = new(log,"PROGRAM");
106 my_xyz_callback xyz = new(log);
107 my_abc_callback abc = new(log);
108
109 initial begin
110 tx.append_callback(abc);
111 tx.append_callback(xyz);
112 tx.start_xactor();
113 #40 ;
114 end
115 endprogram
You could download file vmm_xactor_callback_ex.sv here
|
|
|

|
|
 |
Simulation Log : vmm_xactor_callback
|
|
|

|
|
|
Trace[INTERNAL] on PROGRAM(parent) at 0:
Started
Normal[NOTE] on test(test) at 10:
Ethernet transmit bfm is running
WARNING[FAILURE] on test(test) at 20:
Doing nothing
Normal[NOTE] on test(test) at 30:
Ethernet transmit bfm is running
WARNING[FAILURE] on test(test) at 40:
Corrupting CRC
WARNING[FAILURE] on test(test) at 40:
Corrupting CRC on user request
|
|
|

|
|
|

|
|
|

|
|
|
|
|
|

|
|
|
|
|
|

|
|
Copyright © 1998-2025 |
Deepak Kumar Tala - All rights reserved |
Do you have any Comment? mail me at:deepak@asic-world.com
|
|